What is Activity ?
An activity is an application component that provides a screen with which users can interact in order to do something,What is Fragment ?
A fragment is usually used as part of an activity's user interface and contributes its own layout to the activity. To provide a layout for a fragment, you must implement the onCreateView() callback method, which the Android system calls when it's time for the fragment to draw its layout.
What is an Intent ?
Any android application compromises of one or more Android activities,In order to launch one activity from another activity we have to use app component called as android Intent. An intent is basically an intention to do some action.
It's way to communicate between android components(Not just activities).
It's like a message that Android listens for and react accordingly.
Types of Intent :
Implicit intent used to invoke system components .
(Implicit intents do not directly specify the android component which should be called, its only specifies the action to be performed)
startActivity(intent);
this intents are used in the application itself, wherein one activity can switch to another activity
for example
Intent intent =
new
Intent(
this
, MainActivity.
class
);
startActivity(intent);
to know more about .....
What is content provider ?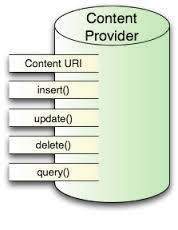
Content provider used to share data between android applications.Shared Preference?
Android provides various method to store data of an application, one of them is called as SharedPreference. SharedPreference will allow the data to store/retrieve in KEY VALUE pair,
In order to get data from sharedPreference we have to call method getSharedPreferences() that returns the shared preference instance pointing to a file that contain value of sharedpreference
In order to get data from sharedPreference we have to call method getSharedPreferences() that returns the shared preference instance pointing to a file that contain value of sharedpreference
SharedPreferences sharedpreferences = getSharedPreferences(MyPREFERENCES, Context.MODE_PRIVATE);
You can save something in the sharedpreference by using shared-preference editor class,
Editor class returns the instance and we will
Editor class returns the instance and we will
Editor editor = sharedpreferences.edit(); editor.putString("key", "value"); editor.commit();
Difference between getContext, getApplicationContext, getBaseContext
|
What is sticky Intent ?
An intent that is used with sticky broadcast, is called as sticky intent. This intent will stick with android system for future broadcast receiver requests.What is relationship between Looper, Handler & MessageQueue in Android?
A
Looper is a message handling loop: it reads and processes items from a MessageQueue . The Looper class is usually used in conjunction with a HandlerThread (a subclass of Thread ).
A
Handler is a utility class that facilitates interacting with a Looper —mainly by posting messages and Runnable objects to the thread's MessageQueue . When a Handler is created, it is bound to a specific Looper (and associated thread and message queue).
In typical usage, you create and start a
HandlerThread , then create a Handler object (or objects) by which other threads can interact with the HandlerThread instance. The Handler must be created while running on the HandlerThread , although once created there is no restriction on what threads can use the Handler 's scheduling methods (post(Runnable) , etc.)
The main thread (a.k.a. UI thread) in an Android application is set up as a looper thread before your application is created.
|
Communication between Fragments
Often you will want one Fragment to communicate with another, for example to change the content based on user event, All Fragment -to-Fragment communication is done through the associated Activity. Two Fragments should never communicate directly.
Here is an example of Fragment to Activity communication:
public class HeadlinesFragment extends ListFragment { OnHeadlineSelectedListener mCallback; // Container Activity must implement this interface public interface OnHeadlineSelectedListener { public void onArticleSelected(int position); } @Override public void onAttach(Activity activity) { super.onAttach(activity); // This makes sure that the container activity has implemented // the callback interface. If not, it throws an exception try { mCallback = (OnHeadlineSelectedListener) activity; } catch (ClassCastException e) { throw new ClassCastException(activity.toString() + " must implement OnHeadlineSelectedListener"); } } ... }
Now the fragment can deliver messages to the activity by calling the
onArticleSelected()
method (or other methods in the interface) using themCallback
instance of the OnHeadlineSelectedListener
interface.
For example, the following method in the fragment is called when the user clicks on a list item. The fragment uses the callback interface to deliver the event to the parent activity.
@Override public void onListItemClick(ListView l, View v, int position, long id) { // Send the event to the host activity mCallback.onArticleSelected(position); }
Implement the Interface
In order to receive event callbacks from the fragment, the activity that hosts it must implement the interface defined in the fragment class.
For example, the following activity implements the interface from the above example.
public static class MainActivity extends Activity implements HeadlinesFragment.OnHeadlineSelectedListener{ ... public void onArticleSelected(int position) { // The user selected the headline of an article from the HeadlinesFragment // Do something here to display that article } }
Deliver a Message to a Fragment
The host activity can deliver messages to a fragment by capturing the
Fragment
instance with findFragmentById()
, then directly call the fragment's public methods.
For instance, imagine that the activity shown above may contain another fragment that's used to display the item specified by the data returned in the above callback method. In this case, the activity can pass the information received in the callback method to the other fragment that will display the item:
public static class MainActivity extends Activity implements HeadlinesFragment.OnHeadlineSelectedListener{ ... public void onArticleSelected(int position) { // The user selected the headline of an article from the HeadlinesFragment // Do something here to display that article ArticleFragment articleFrag = (ArticleFragment) getSupportFragmentManager().findFragmentById(R.id.article_fragment); if (articleFrag != null) { // If article frag is available, we're in two-pane layout... // Call a method in the ArticleFragment to update its content articleFrag.updateArticleView(position); } else { // Otherwise, we're in the one-pane layout and must swap frags... // Create fragment and give it an argument for the selected article ArticleFragment newFragment = new ArticleFragment(); Bundle args = new Bundle(); args.putInt(ArticleFragment.ARG_POSITION, position); newFragment.setArguments(args); FragmentTransaction transaction = getSupportFragmentManager().beginTransaction(); // Replace whatever is in the fragment_container view with this fragment, // and add the transaction to the back stack so the user can navigate back transaction.replace(R.id.fragment_container, newFragment); transaction.addToBackStack(null); // Commit the transaction transaction.commit(); } } }
What is Service class ?
As service is an application component that can perform long-running operations in the background,
It does not provide a user interface. Another application component can start a service, and it continues to run in the background even if the user switches to another application.
public class HelloService extends Service { private static final String TAG = "HelloService"; private boolean isRunning = false; @Override public void onCreate() { Log.i(TAG, "Service onCreate"); isRunning = true; } @Override public int onStartCommand(Intent intent, int flags, int startId) { Log.i(TAG, "Service onStartCommand"); //Creating new thread for my service //Always write your long running tasks in a separate thread, to avoid ANR new Thread(new Runnable() { @Override public void run() { //Your logic that service will perform will be placed here //In this example we are just looping and waits for 1000 milliseconds in each loop. for (int i = 0; i < 5; i++) { try { Thread.sleep(1000); } catch (Exception e) { } if(isRunning){ Log.i(TAG, "Service running"); } } //Stop service once it finishes its task stopSelf(); } }).start(); return Service.START_STICKY; } @Override public IBinder onBind(Intent arg0) { Log.i(TAG, "Service onBind"); return null; } @Override public void onDestroy() { isRunning = false; Log.i(TAG, "Service onDestroy"); } }
0 comments:
Post a Comment